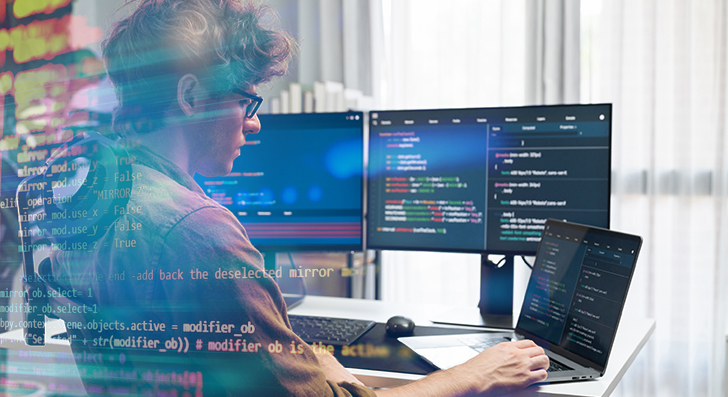
Scalability implies your software can cope with expansion—a lot more customers, extra facts, plus much more targeted traffic—without having breaking. Being a developer, creating with scalability in your mind saves time and stress afterwards. Right here’s a transparent and functional manual to assist you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's strategy from the start. Numerous purposes fail if they expand speedy since the first design and style can’t deal with the additional load. As a developer, you'll want to think early about how your procedure will behave under pressure.
Start off by designing your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. Rather, use modular style and design or microservices. These styles break your app into smaller, independent areas. Each module or services can scale By itself devoid of affecting the whole procedure.
Also, contemplate your databases from working day 1. Will it need to have to take care of a million customers or maybe 100? Pick the appropriate style—relational or NoSQL—based on how your info will increase. Approach for sharding, indexing, and backups early, Even when you don’t require them but.
A different vital position is to stay away from hardcoding assumptions. Don’t create code that only operates underneath present-day conditions. Take into consideration what would come about When your consumer foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that aid scaling, like message queues or event-driven systems. These aid your app manage a lot more requests with no finding overloaded.
Any time you Make with scalability in your mind, you're not just preparing for achievement—you are decreasing long term headaches. A perfectly-prepared program is easier to take care of, adapt, and mature. It’s superior to arrange early than to rebuild afterwards.
Use the best Database
Choosing the suitable database is really a key Component of constructing scalable apps. Not all databases are developed exactly the same, and utilizing the Mistaken one can gradual you down as well as trigger failures as your application grows.
Start off by comprehending your info. Can it be hugely structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is a great match. These are solid with relationships, transactions, and consistency. Additionally they support scaling approaches like go through replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your facts is more adaptable—like consumer exercise logs, item catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally additional easily.
Also, take into account your read and compose styles. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Will you be dealing with a significant write load? Explore databases which will handle large produce throughput, or simply occasion-based mostly facts storage units like Apache Kafka (for short term facts streams).
It’s also smart to Believe forward. You may not need to have State-of-the-art scaling features now, but choosing a database that supports them indicates you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases performance as you grow.
In short, the proper database depends upon your app’s structure, velocity requires, And exactly how you expect it to grow. Take time to pick sensibly—it’ll help you save many issues later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every small hold off provides up. Badly created code or unoptimized queries can slow down general performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by writing clean up, very simple code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple a person will work. Keep your features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or takes advantage of far too much memory.
Following, take a look at your databases queries. These frequently gradual issues down much more than the code itself. Be certain Each and every question only asks for the data you truly need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And stay away from accomplishing too many joins, In particular throughout huge tables.
If you observe the same info staying asked for repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your application much more productive.
Make sure to take a look at with significant datasets. Code and queries that function high-quality with a hundred records may crash after they have to manage one million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when needed. These steps support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional people plus more targeted visitors. If everything goes through one server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of 1 server performing all the work, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When end users request a similar data once more—like an item webpage or perhaps a profile—you don’t really need to fetch it through the database every time. You may serve it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application handle a lot more people, stay quickly, and Get well from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you may need instruments that permit your app develop simply. That’s wherever cloud platforms and containers are available. They give you versatility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic improves, you could add more resources with just a few clicks or automatically making use of automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply companies like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your application and every little thing it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app in between environments, from a notebook for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app works by using various containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In a nutshell, using cloud and container instruments indicates you could scale quickly, deploy conveniently, and Recuperate immediately when troubles happen. If you need your application to expand without the need of limitations, start out utilizing these instruments early. They save time, lessen hazard, and make it easier to stay focused on making, not fixing.
Check All the things
In the event you don’t keep an eye on your software, you won’t know when items go Erroneous. Checking assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it takes for customers to load webpages, how frequently glitches transpire, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, you must get notified right away. This aids you resolve problems quick, often prior to customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new element read more and see a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and details enhance. With out checking, you’ll overlook signs of issues until finally it’s also late. But with the proper applications in place, you continue to be in control.
In short, monitoring helps you maintain your app trusted and scalable. It’s not just about recognizing failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for major businesses. Even smaller apps need to have a strong foundation. By building thoroughly, optimizing wisely, and using the ideal resources, you could Construct applications that grow easily without the need of breaking under pressure. Start off compact, Believe major, and build wise.